RGB Color Layers in p5.js!
A step by step on how to separate an image by the maximum color in it's pixels. The end result will be a function where you can pass either 'RED', 'GREEN', or 'BLUE', as parameters and the output will be either the red, the green or the blue layer of the image used.
Image Loading
Since we are manipulating an image, our first step is going to be using the loadImage() method to upload an image to your sketch and setting up that image as your canvas!
- Use function preload() to load the Image onto your sketch.
- Set the canvas dimentions to the width and height of your image.
- Previous step will make sure image is displayed at the center (since we are setting it up as the canvas itself)
function preload() {
img = loadImage(imglist.starry);
}
function setup(){
createCanvas(img.width, img.height);
pixelDensity(1);
image(img, 0, 0, img.width, img.height);
}
function draw(){
}
ColorLayer( ) Function
Let's create out ColorLayer function. This function should take in a color name as a paramenter. Since this is where we are manipulating the image, we shoud copy and paste the out Basic code from draw into our function. Everything from loadPixels to updatePixels.
function ColorLayer(){
loadPixels();
for (var y = 0; y < height; y++){
for (var x = 0; x < width; x++){
var index = (x + y * width) * 4;
var r = pixels[index+0];
var g = pixels[index+1];
var b = pixels[index+2];
var a = pixels[index+3];
}
}
}
Maximum Color
Our color layers rely on the color with the highest value in that pixel, so lets create a new variable called maxi and the the max() method to determine which of the rgb values is the highest. Since we are analysing each pixel, lets create this variable inside our double for loop.
function ColorLayer(){
loadPixels();
for (var y = 0; y < height; y++){
for (var x = 0; x < width; x++){
var index = (x + y * width) * 4;
var r = pixels[index+0];
var g = pixels[index+1];
var b = pixels[index+2];
var a = pixels[index+3];
var maxi = max([r,g,b]);
}
}
}
Color Options
Our color options are RED, GREEN, and BLUE, so we are going to use those as the valid paramenters for our function. Lets create 3 if statements:
var maxi = max([r,g,b]);
if(color_name == "RED"){
}
if(color_name == "GREEN"){
}
if(color_name == "BLUE"){
}
If the color name is 'BLUE', we are going to keep all the pixels with max of blue and set all of the other ones to white.
if(color_name == "BLUE"){
if(maxi == b){
pixels[index+0] = r;
pixels[index+1] = g;
pixels[index+1] = b;
}
else{
pixels[index+0] = 255;
pixels[index+1] = 255;
pixels[index+1] = 255;
}
}
The same steps are true for 'RED' and 'GREEN'
if(color_name == "RED"){
if(maxi == r){
pixels[index+0] = r;
pixels[index+1] = g;
pixels[index+1] = b;
}
else{
pixels[index+0] = 255;
pixels[index+1] = 255;
pixels[index+1] = 255;
}
if(color_name == "GREEN"){
if(maxi == g){
pixels[index+0] = r;
pixels[index+1] = g;
pixels[index+1] = b;
}
else{
pixels[index+0] = 255;
pixels[index+1] = 255;
pixels[index+1] = 255;
}
Function Call
Our function is ready to go! All we need to do is call it. Let's do that in setup. Remember to pass in a color name!
function preload(){
img = loadImage(imglist.starry);
}
function setup(){
createCanvas(img.width, img.height);
pixelDensity(1);
image(img, 0, 0, img.width, img.height);
ColorLayer("BLUE");
}
function draw(){
}
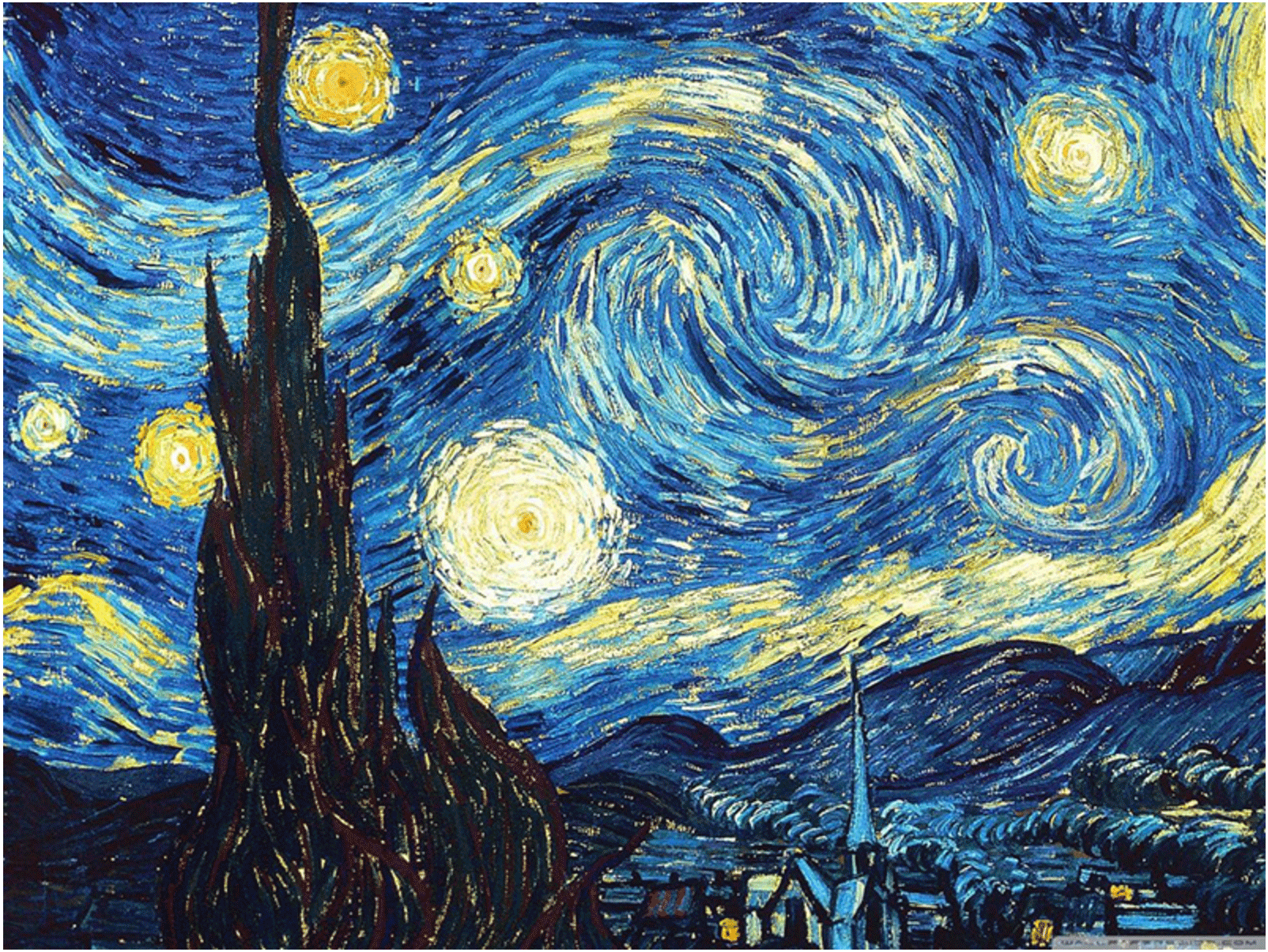